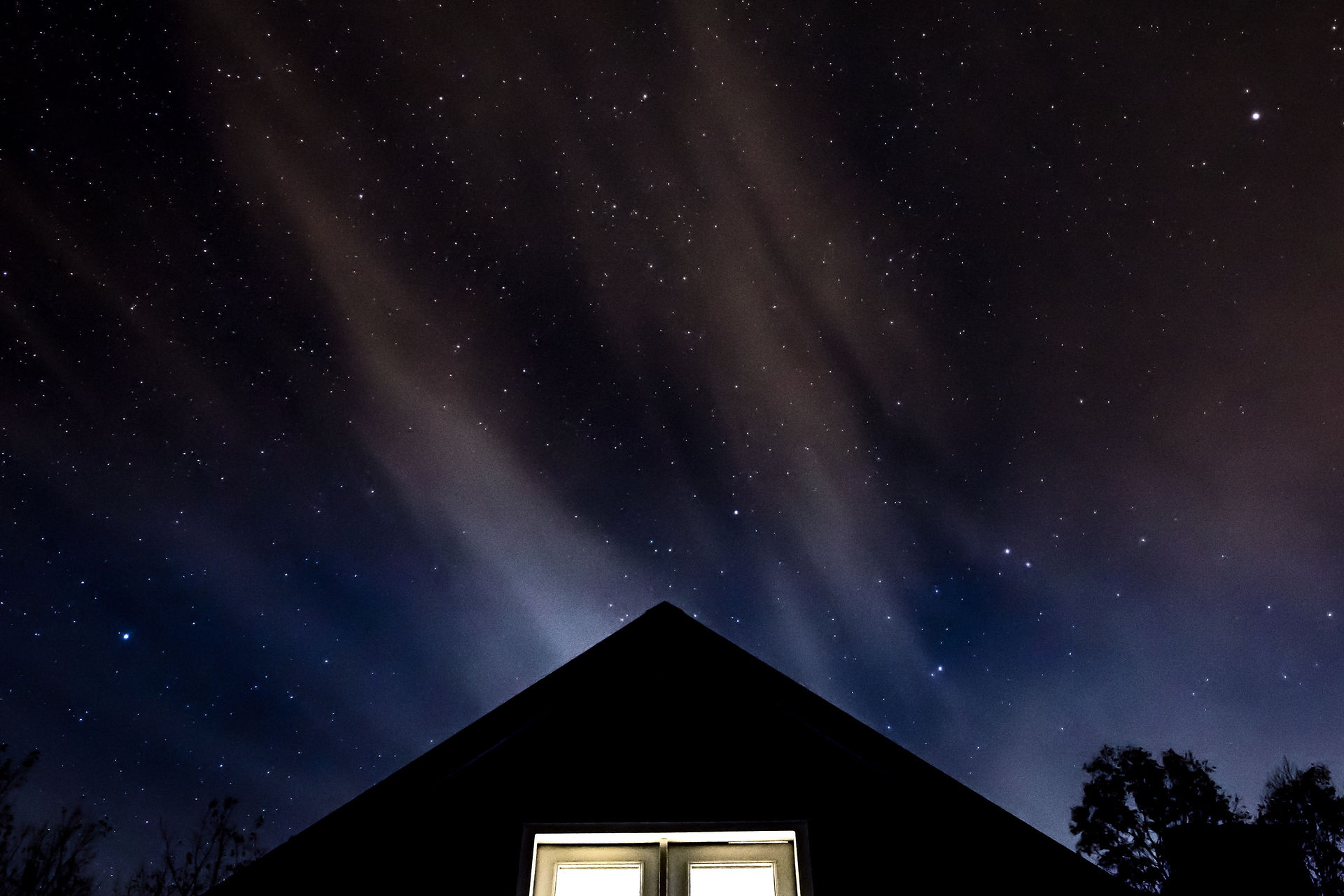
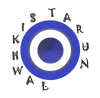
Code
Html & CSS & Js
ProjectsusingHtml & CSS & Js languages with full explanation and with best examples.



Android Studio
Applications in Android Studio with full explanation and with best examples.






C Programs



Hello World Program
#include<stdio.h>
#include<conio.h>
​
void main()
{
printf("Hello World!");
getch();
]



Condition (if/else) Program
#include<stdio.h>
#include<conio.h>
​
void main()
{
int a,b;
printf("Enter values of a and b:");
scanf("%d%d",&a,&b);
if(a>b)
{
printf("A is bigger then B");
}
else
{
printf("B is bigger then A");
}
getch();
}
​



Calculation (+,-,*,/) Program
#include<stdio.h>
#include<conio.h>
​
void main()
{
int a,b;
int add,sub,mul,div;
printf("Enter values of a and b:");
scanf("%d%d".&a,&b);
add=a+b;
sub=a-b;
mul=a*b;
div=a/b;
printf("Addition=%d \n",add);
printf("Subtraction=%d \n",sub);
printf("Multiply=%d \n",mul);
printf("Division=%d \n",div);
getch()
}



Program of Stack
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
void main()
{
clrscr();
int q,top=-1,max=7,a[7],i,ch;
while(1)
{
printf("Enter choice: 1.Push,2.Pop,3.Show,4.Exit:");
scanf("%d",&ch);
switch(ch)
{
case 1:
{
if(top==max-1)
{
printf("Stack is full\n");
}
else
{
top++;
printf("Enter element");
scanf("%d",&a[top]);
}
break;
}
case 2:
{
if(top==-1)
{
printf("Stack is Empty\n");
}
else
{
top--;
}
break;
}
case 3:
{
if(top==-1)
{
printf("Stack is Empty\n");
}
else
{
printf("Stack elements=\n");
for(i=top;i>=0;--i)
{
printf("%d\n",a[i]);
}
}
break;
}
case 4:
{
printf("Exit");
exit(0);
}
default :
{
printf("Enter correct :");
}
}
}
getch();
}



Program of Queue
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
void main()
{
clrscr();
int x,i,ch,front=-1,rare=-1,queue[7],max=7;
while(1)
{
printf("Enter Choice: 1.Push,2.Pop,3.Show,4.Exit");
scanf("%d",&ch);
switch(ch)
{
case 1:
{
if(rare==max-1)
{
printf("Queue is full.\n");
}
else
{
if(front==-1)
{
front=0;
}
rare=rare+1;
printf("Enter Element:");
scanf("%d",&x);
queue[rare]=x;
}
break;
}
case 2:
{
if(front==-1 || front>rare)
{
printf("Queue is Empty.\n");
}
else
{
printf("Element deleted: %d\n",queue[front]);
front=front+1;
}
break;
}
case 3:
{
if(front==-1 || front>rare)
{
printf("Queue is Empty.\n");
}
else
{
printf("Queue Elements:\n");
for(i=front;i<=rare;i++)
{
printf("%d\t",queue[i]);
}
printf("\n");
}
break;
}
case 4:
{
printf("Exit..");
exit(0);
}
default :
{
printf("Enter Correct Choice->");
break;
}
}
}
getch();
}



C++ Programs



Hello World Program
#include<iostream.h>
#include<stdio.h>
#include<conio.h>
​
void main()
{
cout<<"Hello World!";
getch();
]



Condition (if/else) Program
#include<iostream.h>
#include<stdio.h>
#include<conio.h>
​
void main()
{
int a,b;
cout<<"Enter values of a and b:";
cin>>a>>b;
if(a>b)
{
cout<<"A is bigger then B";
}
else
{
cout<<"B is bigger then A";
}
getch();
}



Calculation (+,-,*,/) Program
#include<iostream.h>
#include<stdio.h>
#include<conio.h>
​
void main()
{
int a,b;
int add,sub,mul,div;
cout<<"Enter values of a and b:";
cin>>a>>b;
add=a+b;
sub=a-b;
mul=a*b;
div=a/b;
cout<<"Addition ="<<add;
cout<<"Subtraction="<<sub;
cout<<"Multiply="<<mul;
cout<<"Division="<<div;
getch()
}